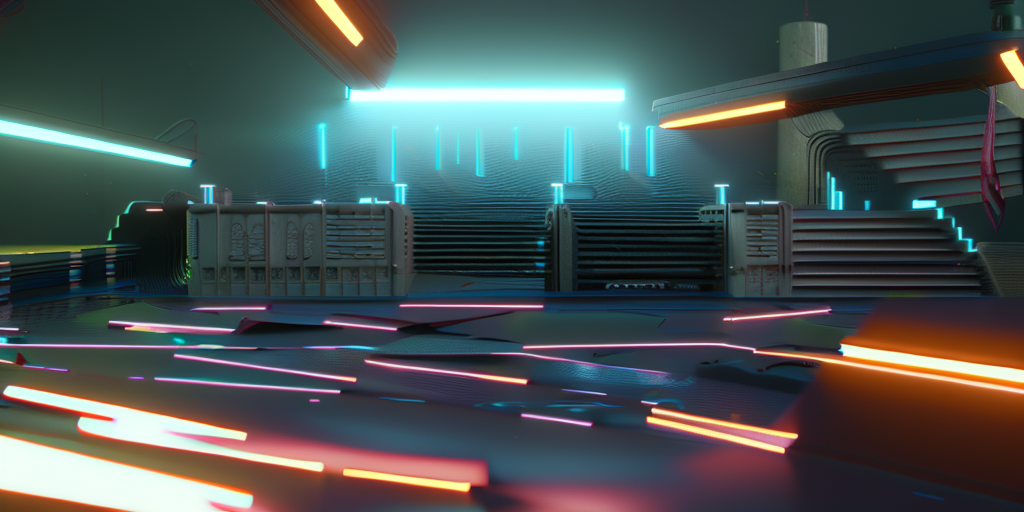
Hey there, folks!
Today, I'm gonna walk you through the process of building a kickass blog website using the ever-popular Django framework, step by step.
Now, if you're new to Django, no worries, my friend! It's a Python-based web framework that's known for being super easy to learn. But before we dive into the nitty-gritty, you'll need to have some prerequisites down pat to follow along with ease. These include a solid understanding of front-end basics (that means knowing the basics of HTML and CSS, folks) and a good grasp of Python basics as well.
Ready to rock and roll? Let's get started!
Alright, let's get this party started by installing Django on your system. But hold on, if you've already got Django installed, you can skip this part and jump straight into the action.
For Windows users, you'll want to open up your trusty command prompt (CMD) and type in the following command:
pip install django
On the other hand, if you're using either a MAC or Linux, simply open up your command line and type in the following command:
pip3 install django
And voila! With just a few keystrokes, you're now ready to begin building your Django-powered website.
Alright, let's kick off our project by taking the first step. First, create a new folder on your desktop and give it a name. I prefer naming mine 'BlogProject'. Next, open up your command line (CMD) and navigate to the newly created folder using the following commands:
cd Desktop
cd BlogProject
Once you're in the folder, it's time to create a Django project called 'blog'. Run the command below to do so:
django-admin startproject blog
After that, navigate into the 'blog' folder and create a new app called 'articles' using the following commands:
cd blog
python manage.py startapp articles
If you're using a Mac or Linux, be sure to type 'python3' instead of 'python'. Once that's done, we can proceed with the migration process:
python manage.py migrate
Finally, let's start the server using the following command:
You'll be given a URL, so copy and paste it into a new tab in your browser. If everything has been set up correctly, you should see a rocket icon. (Your URL will look something like this: http://127.0.0.1:8000/ )
Now, let's open up our IDE and navigate to the project (blog). Once there, open up the 'settings.py' file inside the 'blog' folder and add the name of the app we just created ('articles') to the INSTALLED_APPS list.
Alright, it's time to move onto the next step: creating the database models. Since we're building a blog, we need three main things: an author, a title, and some body text. To accommodate this, we'll create three fields in our model.
To allow authors to write multiple articles (creating a many-to-one relationship), we'll set the author field as a ForeignKey. Open up your models.py file and paste in the following code:
from django.contrib import admin
from .models import Article
admin.site.register(Article)
There you have it! Your models are now set up and ready to go.
In this step, we're going to set up the admin panel for our blog. First, open your command line and navigate to the 'blog' folder. Then, run the following commands:
python manage.py makemigrations articles
python manage.py migrate articles
If you're on a Mac or Linux, use python3
instead of python
.
Now, let's create a user to log in to the admin panel. Run the following command:
python manage.py createsuperuser
Enter your desired username, email, and password, and your superuser will be created.
With that out of the way, let's start up the server:
python manage.py runserver
Copy the URL that's displayed and paste it into a new tab in your browser. Add '/admin' to the end of the URL, and you should be taken to the admin login page.
Log in using the username and password you just created, and you should see the admin interface. From there, you can click on 'Articles' and add new articles to your blog. Give it a try and save a few articles!
Let's move on to setting up the URLs. Open the urls.py file in the project folder and make the following changes to the code at the bottom portion of that file:
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('', include('articles.urls')),
]
Note that we have mentioned the articles.urls
file, but that doesn't exist yet. Before that, we need to create the views. Open views.py and create your views there:
from django.views.generic import ListView
from .models import Article
class ArticleListView(ListView):
model = Article
template_name = 'home.html'
Now, let's create our templates folder. Create a new folder called templates
inside the project folder. Inside the templates
folder, create a new file and name it home.html
.
we need to configure the templates in the settings.py file. In the TEMPLATES list, we have to specify the path of our templates folder. To do that, add the following code to the list:
'DIRS': [os.path.join(BASE_DIR, 'templates')],
This code will look for the templates folder in the base directory of our project.
Then, create a new HTML file called 'home.html' inside the templates folder, and add the following code to display the articles fetched from the database using a for loop.
Note that this tutorial focuses on the backend rather than the design, so we won't be discussing the HTML part in detail.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>My Blog</title>
</head>
<body>
<header>
<div class="container">
{% block content %}
{% for article in object_list %}
<div class="article">
<h3><a href="">{{ article.title }}</a></h3>
<p>{{ article.body }}</p>
</div>
{% endfor %}
{% endblock %}
</div>
</header>
</body>
</html>
Note that everything that needs to be fetched from the database is written inside the {% block content %} section, which ends at {% endblock content %}. All the articles are fetched one by one using a 'for loop'.
Alright, let's dive into including static files in our blog. To begin with, we need to create a new folder called 'static' inside the blog folder, which happens to be the project folder, not the inner one.
Now comes the linking part. We need to link this folder in the settings file. So, let's open the settings.py file and insert the following code snippet at the bottom, right below the already existing STATIC_URL part:
STATICFILES_DIRS = [os.path.join(BASE_DIR, 'static')]
Great! Now, it's time to add some styling to our blog. Create a new folder called 'css' inside the static folder. Within 'css', create a new file named 'style.css' and add your CSS styles as per your liking. For the sake of simplicity, I'll be adding only a few styles to my blog. Here's what I've pasted into my style.css file:
body{
background-color: #eee;
color: #222;
}
Next, we need to link the CSS file in our home.html file. Open up the home.html file and insert the following line of code between the <head> </head> tags:
<link rel="stylesheet" href="{% static 'css/style.css' %}">
Take note that the href part differs from what we usually do with HTML and CSS. Here, we have to link it in the Django way.
Now, let's mention in home.html that we are using static files. Simply paste the following line at the top of the home.html file:
{% load staticfiles %}
If you're using the latest version of Django, then use {% load static %} instead of {% load staticfiles %}, otherwise, an error may occur.
That's all there is to it! You're now free to modify and customize your blog however you like.
Alright, let's dive into Step 7 where we'll learn how to set up unique URLs for each article on our blog. This way, when users click on an article on the home page, they'll be directed to a separate page containing the article in detail.
Setting this up is actually quite easy. We'll need to create a separate template file and view for each article. First, let's open up views.py and add the following class:
class ArticleDetailView(DetailView):
model = Article
template_name = 'detail.html'
Don't forget to import DetailView on the first line, like so:
from django.views.generic import ListView, DetailView
Next, we'll create a new file for the detailed article. Go ahead and create a new file called detail.html
inside the templates folder. Then, paste in the following code snippet:
{% load static %}
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<link rel="stylesheet" href="{% static 'css/style.css' %}">
<title>My Blog</title>
</head>
<body>
<header>
<h1> <a href="">My Personal Blog</a> </h1>
</header>
<div class="container">
{% block content %}
<div class="details">
<h2>{{ article.title }}</h2>
<p>{{ article.body }}</p>
</div>
{% endblock content %}
</div>
</body>
</html>
Note that we added {{ article.title }}
and {{ article.body }}
here. These will fetch our article’s title and body part into the page.
Now, we have to mention the URL to urls.py
. Open up urls.py
in the articles folder and add the following line of code as the path:
path('article/<int:pk>', views.ArticleDetailView.as_view(), name='detail'),
The int.pk
in this file indicates the primary key. pk
stands for the primary key here. The database automatically gives a unique ID for each article, and that unique ID is also called the primary key.
Lastly, let's open up home.html
and add the href
link to the h3
article heading. So, modify the line that contains the <h3>
tag inside the class article like this:
<h3><a href="{% url 'detail' i.pk %}">{{ i.title }}</a></h3>
That’s it! Our blog is ready to run.
That’s it! Our blog is ready to run. Your server should be automatically reloaded by now. You're all set to modify and customize your blog as you like.
Write a comment ...